Word2Vec Using Gensim
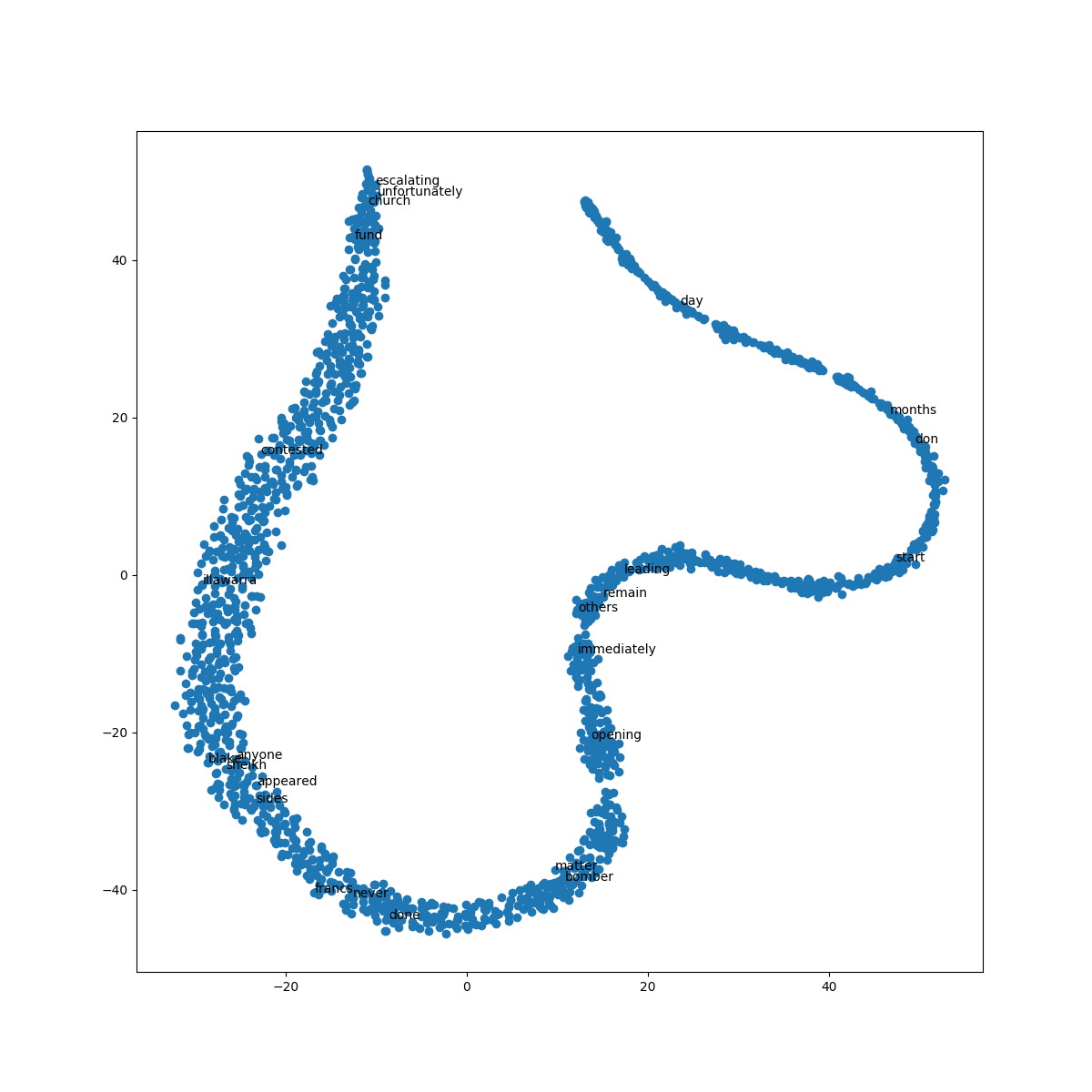
บทความโดย อ.ดร.ณัฐโชติ พรหมฤทธิ์ และอ.ดร.สัจจาภรณ์ ไวจรรยา
ภาควิชาคอมพิวเตอร์
คณะวิทยาศาสตร์
มหาวิทยาลัยศิลปากร
จากที่เราได้ทดลองสร้าง Word2Vec Model แบบ Skip Gram โดยใช้ Keras ของ Tensorflow กันมาแล้ว ซึ่งวิธีการก่อนหน้านี้ เราได้เห็นกระบวนการในการสร้างโดยละเอียดที่เราทำทีละขั้นตอน และสร้าง Model ขึ้นเองเพื่อ Train Word2Vec Model ซึ่งพบปัญหาว่าถ้าข้อความยาวมาก ๆ หรือ Dataset มีขนาดใหญ่ Model จะทำงานช้า ถึงช้ามาก
ในบทความนี้ เราจะใช้ Gensim ซึ่งเป็น open source Python Library ในกลุ่ม NLP ที่ Focus ด้าน Topic Modeling.
ก่อนอื่นเราจะติดตั้ง Library ที่ต้องใช้งาน ซึ่งบทความนี้เราจะลองใช้ตัวตัดคำของ PythaiNLP
!pip install pythainlp
import pandas as pd
import re
from pythainlp.tokenize import word_tokenize
import numpy as np
from gensim.models import Word2Vec
อ่าน file test.csv จาก Dataset ที่เตรียมไว้ ซึ่งเป็นชุดข้อมูลที่มีการเผยแพร่ไว้จากแหล่งข้อมูลที่รวบรวมข้อความสำหรับการทำ Sentiment Analysis
comments = []
with open("test.csv") as f:
for line in f:
comments.append(line.strip())
- ดูจำนวนความคิดเห็น ซึ่งมีทั้งหมด 2674 ข้อความ
len(comments)
- แปลงข้อความเป็น Data Frame
df = pd.DataFrame({ "comments": comments })
df.head()
- ทำความสะอาดข้อความในเบื้องต้น ซึ่งในที่นี้จะทดลองโดยเลือกเฉพาะข้อความภาษาไทย และตัวเลข
df.replace(r'[^ก-๙0-9]','',regex=True, inplace = True)
df.head()
- ดึงข้อความออกจาก Data Frame
sentences = df.comments.values
sentences.shape
sentences[0:5]
- นำข้อความมาตัดคำ
tsentences = []
for st in sentences:
tokens = word_tokenize(st.strip())
tsentences.append(tokens)
tsentences[0:5]
- กำหนด dimension
dimension = 128
- สร้าง Word2Vec โดยกำหนดพารามิเตอร์ ต่างๆ ที่ต้องการ ซึ่งรายละเอียดของพารามิเตอร์ สามารถดูได้จาก https://radimrehurek.com/gensim/auto_examples/tutorials/run_word2vec.html#sphx-glr-auto-examples-tutorials-run-word2vec-py
w2v_model = Word2Vec(tsentences, min_count=1, vector_size=dimension, workers=6, sg=1, epochs=100)
- ดูข้อมูลของ Model
print(w2v_model)
จะพบว่าจากข้อมูลที่เรานำมาใช้จะเป็น
Word2Vec(vocab=7216, size=128, alpha=0.025)
- Save Model
w2v_model.save('w2v_model.bin')
- load Model
new_model = Word2Vec.load('w2v_model.bin')
หลังจากที่ Load Model แล้ว เราสามารถดูข้อมูลของคำใน Word2Vec ได้โดยใช้ <self>.wv.<function>
- ลองดู Word Similarity
new_model.wv.similarity('อร่อย', 'เยี่ยม')
- ลองดูคำที่มีค่า Similarity สูงสุด 5 อันดับ
new_model.wv.most_similar(positive=['ชาบู'], topn=5)
- ดึงคำทั้งหมดจาก Model ออกมา
#words = list(new_model.wv.vocab)
words = list(new_model.wv.index_to_key)
- ดึง vector ของคำจาก Model ออกมา
vectors = list(new_model.wv.vectors)
จากนั้นเราก็สามารถนำคำ และ Vector ของคำไปสร้างเป็น File เพื่อ Plot บน https://projector.tensorflow.org/
import csv
with open('w2vG.tsv', 'w') as out_file:
tsv_writer = csv.writer(out_file, delimiter='\t')
for v in vectors:
tsv_writer.writerow(v)
with open('labelG.tsv', 'w') as out_file:
tsv_writer = csv.writer(out_file, delimiter='\t')
for word in words:
tsv_writer.writerow([word])